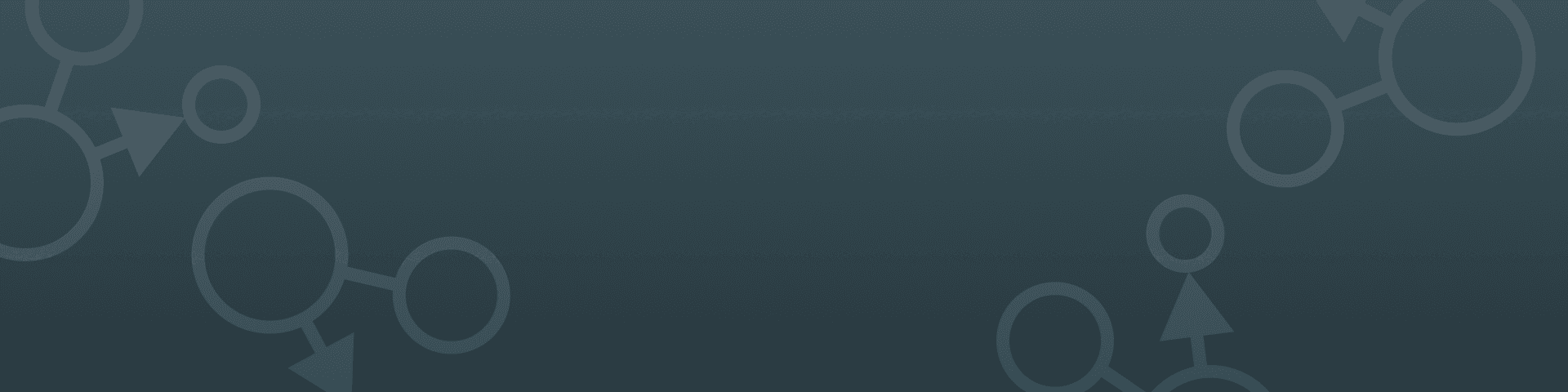
Practice coding
Not sure if you're ready for our interview? Try this problem to benchmark yourself.
Before you start
This is not our real interview problem, but it is similar and representative.
Very few people will finish the full problem. You do not need to complete every step to pass our interview.
Hacky solutions are OK. All else equal, optimize for speed.
You may Google small questions or use IDE features, but not use an LLM/other gen AI (including copilot).
Before you scroll down any further:
Have an IDE open
Refresh on how to take console I/O. Make sure you have a working console (e.g. if you're using JavaScript, you'll want to have Node running).
Set a timer for 25 minutes. Start it before you begin reading the prompt.
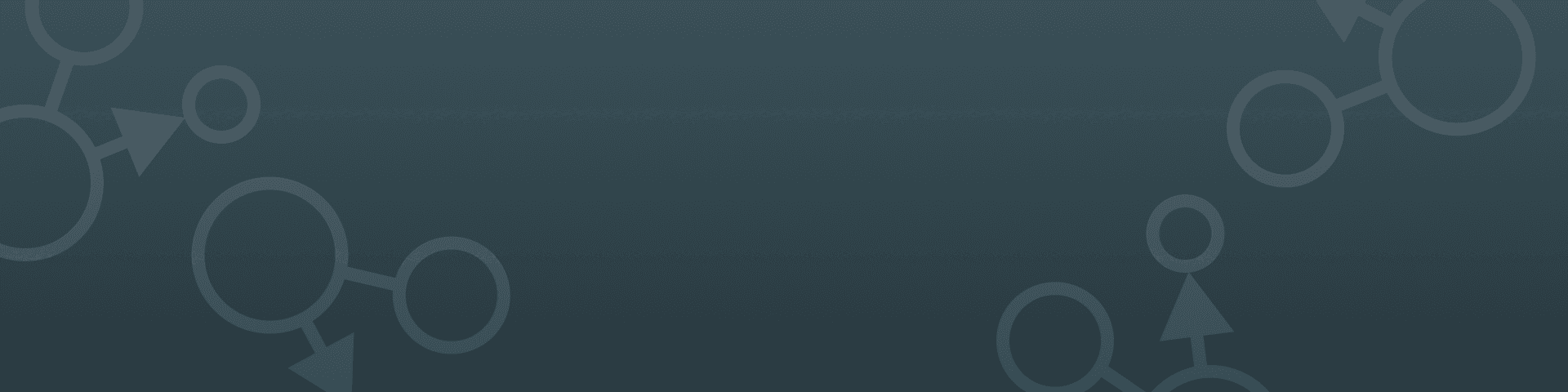
(This space left blank so you can scroll down when you're ready.)
The problem
In this problem, we'll build a simplified version of the game Minesweeper in the command line.
This is a puzzle game for one player. It's played on an NxN grid, where some squares in the grid are marked as "mines". Normally, these mines are kept secret from the player, because the objective of the game is to discover the location of every mine without revealing them. In this exercise, we'll keep the mines revealed for simplicity.
On each input, the player may select any square on the grid, and one of two things happens:
If the square they selected is a mine, they lose immediately.
Otherwise, the square changes to display the number of adjacent mines (from 0 to 8). This number remains displayed in that grid square until the end of the game.
The player wins when they have revealed all squares that are NOT mines.
Step 1
Create a data structure to store the state of a game. Create a 4x4 board and place mines in the first two places in the third column.
Print the board to the console, representing an empty square with a dash (-) character and a mine with an asterisk (*) character. Your output should look like the image shown.
Step 2
Create a function to reveal a square on the board.
This function should take two arguments, a row and a column, and reveal the selected square according to the rules of Minesweeper:
If the selection is a mine, print "you lose" to the player.
If the selection is not a mine, change that square to display the number of mines adjacent to it (including diagonally, so the value may range from 0 to 8).
Test your function by revealing the first, third, and fourth squares in the second column, then print the board. Your output should look like the image shown.
Step 3
Take user input.
Prompt the user in the command line to enter a (1-indexed) row and column, separated by a comma. For example, if the user enters 2,3
, reveal the second row and third column.
If the user's input is invalid, do nothing and prompt them for a new one.
If the user's input is valid, reveal the square using the function from the previous step, print the board, and (if the player did not lose) prompt for the next input.
Remove the test code from the previous step, then test your new code by inputting the following via the newly-implemented input prompt. Check your result against the image.
1,1
2,4
2,3
Step 4
Add a win check
After a player's move, check whether every square that is not a mine has been revealed. If so, print "you win" after the player's move and do not prompt them for another move.
Test your code by increasing the number of mines on your board to cover all but (2,3) and (1,1), then select those two squares by entering 2,3 and 1,1. Check your result against the image.
Step 5
Add flood-revealing
Modify your square-revealing function so that when a player reveals a square with a 0 (that is, with no adjacent mines), all squares adjacent to that square are also revealed. If those squares also have no adjacent mines, repeat the same process for those squares as well, until all 0's in a contiguous region (and all cells adjacent to one of those 0's) are revealed.
(Note that this can never reveal a mine, so it can never cause the player to lose. It may cause a player to win, however.)
Test your code by returning to the original board (4x4 with mines in the first two squares of the third column), then entering 4, 1. Your output should look like the image shown.
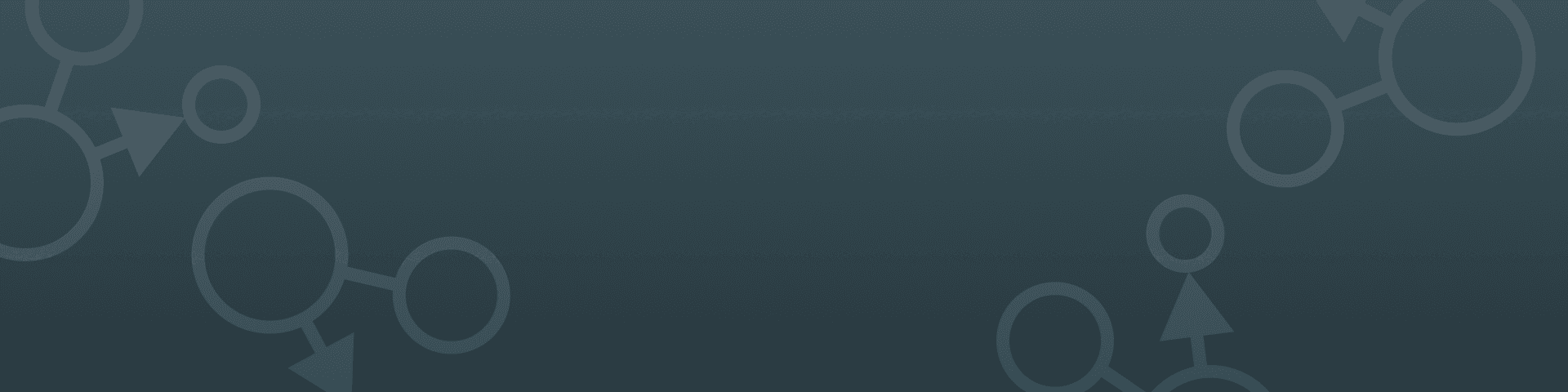