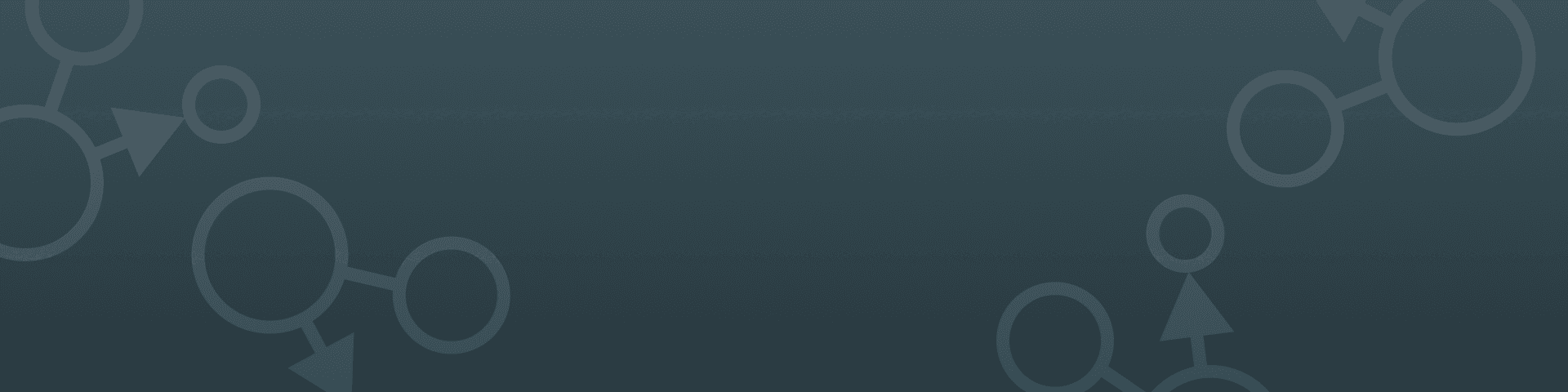
Interview prep
Taking our interview? Here's what to expect.
What you need
A working webcam. Our interview is done via video call, and you'll need to be on video for most of the call. (We know this isn't ideal for anxious people, but it's a practical necessity.)
A dev environment with a command line or console I/O ready to go. In particular, if you're using JS, make sure you have Node set up and can take console I/O in it.
The ability to share your screen. During the coding section of our interview, we'll ask you to share it as you write your code.
Structure
The interview is a 90-minute video call with one of our engineers.
We don't expect you to know any specific languages or technologies, except for some JS-specific front-end questions.
You can use the tools you're most comfortable with for coding, including your usual language and IDE (within reason, you probably shouldn't do it in brainfuck).
Content
The interview is divided into three sections: live coding, conceptual questions, and system design. Scroll down a bit for more details on each.
You are not expected to fully complete the coding exercise, or to know every question we ask. Our interview is meant to be broad enough to capture different strengths - good coding plus two other good or one other excellent section is usually sufficient.
Section details
Scoring
Each section is scored on a mix of objective progress and subjective impressions. We normalize these scores between interviewers, so that you don't get an advantage or disadvantage based on luck of the draw.
We also don't tell interviewers about your background ahead of time. They score you based on what they see.
Feedback
We always give candid feedback, no matter the result. Regardless of whether you passed our interview, we'll offer any suggestions we can on how to prep for your next interview.
Scroll down for a detailed example.